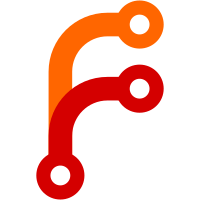
Some checks are pending
/ build (push) Waiting to run
Signed-off-by: Minecon724 <git@m724.eu>
42 lines
1.3 KiB
Java
42 lines
1.3 KiB
Java
/*
|
|
* Copyright (c) 2025 blog-software-java developers
|
|
* blog-software-java is licensed under the GNU General Public License. See the LICENSE.md file
|
|
* in the project root for the full license text.
|
|
*/
|
|
|
|
package eu.m724.blog.compress;
|
|
|
|
import org.apache.commons.compress.compressors.CompressorException;
|
|
import org.apache.commons.compress.compressors.CompressorStreamFactory;
|
|
|
|
import java.io.IOException;
|
|
import java.nio.file.*;
|
|
|
|
public class FileCompressor {
|
|
private final String algorithm;
|
|
|
|
public FileCompressor(String algorithm) {
|
|
this.algorithm = algorithm;
|
|
}
|
|
|
|
public void compress(Path source) throws IOException, CompressorException {
|
|
var destination = source.resolveSibling(source.getFileName() + "." + algorithm);
|
|
compress(source, destination);
|
|
}
|
|
|
|
public void compress(Path source, Path destination) throws IOException, CompressorException {
|
|
if (Files.exists(destination))
|
|
throw new FileAlreadyExistsException(destination.toString());
|
|
|
|
try (
|
|
var outputStream = new CompressorStreamFactory()
|
|
.createCompressorOutputStream(algorithm, Files.newOutputStream(destination))
|
|
) {
|
|
Files.copy(source, outputStream);
|
|
}
|
|
}
|
|
|
|
public String getAlgorithm() {
|
|
return algorithm;
|
|
}
|
|
}
|