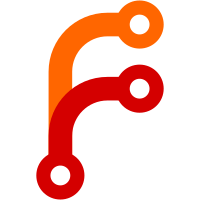
Some checks are pending
/ build (push) Waiting to run
- Replace Git dependency in `Site` with `Path` for config files - Refactor `Server` initialization and add browser-opening functionality - Introduce `BlogBuilder` for blog building logic separation - Simplify `Main` by delegating build and server logic to `BlogBuilder` and `Server` - Add CLI enhancements with new options and improved readability Signed-off-by: Minecon724 <git@m724.eu>
71 lines
2.1 KiB
Java
71 lines
2.1 KiB
Java
package eu.m724.blog;
|
|
|
|
import com.sun.net.httpserver.HttpExchange;
|
|
import com.sun.net.httpserver.HttpHandler;
|
|
import com.sun.net.httpserver.HttpServer;
|
|
|
|
import java.io.IOException;
|
|
import java.net.InetSocketAddress;
|
|
import java.nio.charset.StandardCharsets;
|
|
import java.nio.file.Files;
|
|
import java.nio.file.Path;
|
|
|
|
public class Server implements HttpHandler {
|
|
private final Path webroot;
|
|
private InetSocketAddress listenAddress;
|
|
|
|
public Server(Path webroot, InetSocketAddress listenAddress) {
|
|
this.webroot = webroot;
|
|
this.listenAddress = listenAddress;
|
|
}
|
|
|
|
public Server(Path webroot) {
|
|
this(webroot, new InetSocketAddress("localhost", 0));
|
|
}
|
|
|
|
public void start() throws IOException {
|
|
var server = HttpServer.create(listenAddress, 0);
|
|
server.createContext("/", this);
|
|
server.start();
|
|
|
|
System.out.println("Server started on http:/" + server.getAddress());
|
|
|
|
this.listenAddress = server.getAddress();
|
|
}
|
|
|
|
public void openBrowser() {
|
|
try {
|
|
var process = Runtime.getRuntime().exec(new String[] { "xdg-open", "http://" + listenAddress.getHostString() + ":" + listenAddress.getPort() });
|
|
var code = process.waitFor();
|
|
if (code != 0) {
|
|
throw new Exception("Exit code " + code);
|
|
}
|
|
System.out.println("Opened browser");
|
|
} catch (Exception e) {
|
|
System.out.println("Failed to open browser: " + e);
|
|
}
|
|
}
|
|
|
|
@Override
|
|
public void handle(HttpExchange exchange) throws IOException {
|
|
var path = webroot.resolve(exchange.getRequestURI().getPath().substring(1));
|
|
|
|
if (Files.isDirectory(path)) {
|
|
path = path.resolve("index.html");
|
|
}
|
|
|
|
var code = 404;
|
|
var content = "Not found".getBytes(StandardCharsets.UTF_8);
|
|
|
|
if (Files.isRegularFile(path)) {
|
|
code = 200;
|
|
content = Files.readAllBytes(path);
|
|
}
|
|
|
|
exchange.sendResponseHeaders(code, content.length);
|
|
|
|
var body = exchange.getResponseBody();
|
|
body.write(content);
|
|
body.close();
|
|
}
|
|
}
|