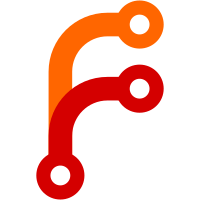
- Added Javadoc comments to `Site`, `Server`, `Feed`, `BlogBuilder`, `TemplateRenderer`, and `Post` classes. - Documented constructors, methods, and class-level descriptions. - Enhanced clarity around parameters, return values, and exceptions. Signed-off-by: Minecon724 <git@m724.eu>
112 lines
4.3 KiB
Java
112 lines
4.3 KiB
Java
package eu.m724.blog;
|
|
|
|
import eu.m724.blog.data.Post;
|
|
import eu.m724.blog.data.Site;
|
|
import io.pebbletemplates.pebble.PebbleEngine;
|
|
import io.pebbletemplates.pebble.extension.AbstractExtension;
|
|
import io.pebbletemplates.pebble.extension.Function;
|
|
import io.pebbletemplates.pebble.loader.FileLoader;
|
|
import io.pebbletemplates.pebble.template.EvaluationContext;
|
|
import io.pebbletemplates.pebble.template.PebbleTemplate;
|
|
|
|
import java.io.IOException;
|
|
import java.io.StringWriter;
|
|
import java.nio.file.Path;
|
|
import java.util.ArrayList;
|
|
import java.util.List;
|
|
import java.util.Map;
|
|
|
|
/**
|
|
* The {@code TemplateRenderer} class is responsible for rendering dynamic HTML templates
|
|
* using the Pebble templating engine.
|
|
*/
|
|
public class TemplateRenderer {
|
|
private final PebbleTemplate indexTemplate, articleTemplate;
|
|
|
|
/**
|
|
* Constructs a TemplateRenderer instance for rendering templates from the specified directory.
|
|
*
|
|
* @param templateDirectory the root directory containing the template files
|
|
*/
|
|
public TemplateRenderer(Path templateDirectory) {
|
|
var loader = new FileLoader();
|
|
loader.setPrefix(templateDirectory.toString());
|
|
loader.setSuffix(".html");
|
|
|
|
var pebbleEngine = new PebbleEngine.Builder()
|
|
.loader(loader)
|
|
.extension(new AbstractExtension() {
|
|
@Override
|
|
public Map<String, Function> getFunctions() {
|
|
return Map.of(
|
|
"static", new Function() {
|
|
@Override
|
|
public List<String> getArgumentNames() {
|
|
return List.of("path");
|
|
}
|
|
|
|
@Override
|
|
public Object execute(Map<String, Object> args, PebbleTemplate self, EvaluationContext context, int lineNumber) {
|
|
return "/static/" + args.get("path"); // TODO for more advanced stuff
|
|
}
|
|
},
|
|
"asset", new Function() {
|
|
@Override
|
|
public List<String> getArgumentNames() {
|
|
return List.of("path");
|
|
}
|
|
|
|
@Override
|
|
public Object execute(Map<String, Object> args, PebbleTemplate self, EvaluationContext context, int lineNumber) {
|
|
return "/assets/" + args.get("path"); // TODO for more advanced stuff
|
|
}
|
|
}
|
|
);
|
|
}
|
|
})
|
|
.build();
|
|
|
|
this.indexTemplate = pebbleEngine.getTemplate("index_template");
|
|
this.articleTemplate = pebbleEngine.getTemplate("article_template");
|
|
}
|
|
|
|
/**
|
|
* Renders the index page using this template.
|
|
*
|
|
* @param site the {@link Site} this index is for
|
|
* @param posts the {@link Post}s to be included in the index page
|
|
* @return the rendered index HTML page as a string
|
|
* @throws IOException if an error occurs during the template evaluation
|
|
*/
|
|
public String renderIndex(Site site, ArrayList<Post> posts) throws IOException {
|
|
Map<String, Object> context = Map.of(
|
|
"site", site,
|
|
"articles", posts
|
|
);
|
|
|
|
var writer = new StringWriter();
|
|
indexTemplate.evaluate(writer, context);
|
|
|
|
return writer.toString();
|
|
}
|
|
|
|
/**
|
|
* Renders the content of a post using this template.
|
|
*
|
|
* @param site the {@link Site} containing the post
|
|
* @param post the {@link Post} to be rendered
|
|
* @return the rendered post HTML page as a string
|
|
* @throws IOException if an error occurs during template evaluation
|
|
*/
|
|
public String renderPost(Site site, Post post) throws IOException {
|
|
Map<String, Object> context = Map.of(
|
|
"site", site,
|
|
"article", post
|
|
);
|
|
|
|
var writer = new StringWriter();
|
|
articleTemplate.evaluate(writer, context);
|
|
|
|
return writer.toString();
|
|
}
|
|
}
|