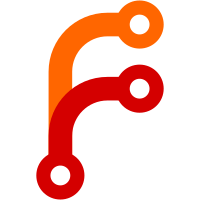
- Added hover and click actions to chat commands for better interaction. - Enhanced error and confirmation messages for clarity. - Introduced validation for room IDs and improved handling of invalid IDs. Signed-off-by: Minecon724 <git@m724.eu>
106 lines
3.2 KiB
Java
106 lines
3.2 KiB
Java
/*
|
|
* Copyright (C) 2025 Minecon724
|
|
* Tweaks724 is licensed under the GNU General Public License. See the LICENSE.md file
|
|
* in the project root for the full license text.
|
|
*/
|
|
|
|
package eu.m724.tweaks.module.chat;
|
|
|
|
import net.md_5.bungee.api.ChatColor;
|
|
import org.bukkit.Bukkit;
|
|
import org.bukkit.configuration.file.YamlConfiguration;
|
|
import org.bukkit.plugin.Plugin;
|
|
|
|
import java.io.File;
|
|
import java.io.IOException;
|
|
import java.util.UUID;
|
|
|
|
public class ChatRoomLoader {
|
|
private static File chatRoomsDir;
|
|
|
|
static void init(Plugin plugin) {
|
|
chatRoomsDir = new File(plugin.getDataFolder(), "storage/rooms");
|
|
chatRoomsDir.mkdirs();
|
|
}
|
|
|
|
/**
|
|
* Get the file of persistent storage of a chat room
|
|
* @return the file or null if ID is invalid
|
|
*/
|
|
static File getFile(String id) {
|
|
if (validateId(id) != 0)
|
|
return null;
|
|
|
|
return new File(chatRoomsDir, id + ".yml");
|
|
}
|
|
|
|
/**
|
|
* Checks if an id is valid and returns why it's not
|
|
*
|
|
* @return 0 if ok<br>
|
|
* 1 if too short (<2 chars)
|
|
* 2 if too long (>20 chars)
|
|
* 3 if not lowercase
|
|
* 4 if not alphanumeric
|
|
*/
|
|
static int validateId(String id) {
|
|
if (id.length() < 2) {
|
|
return 1;
|
|
} else if (id.length() > 20) {
|
|
return 2;
|
|
} else if (!id.equals(id.toLowerCase())) {
|
|
return 3;
|
|
} else if (!id.chars().allMatch(Character::isLetterOrDigit)) {
|
|
return 4;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
/**
|
|
* Loads a chat room from disk<br>
|
|
* A lowercase, alphanumeric id is expected
|
|
*
|
|
* @param id the id of the chat room
|
|
* @return the chat room or null if no such chat room
|
|
*/
|
|
static ChatRoom load(String id) {
|
|
File chatRoomFile = getFile(id);
|
|
if (chatRoomFile == null || !chatRoomFile.exists())
|
|
return null;
|
|
|
|
YamlConfiguration configuration = YamlConfiguration.loadConfiguration(chatRoomFile);
|
|
|
|
ChatRoom chatRoom = new ChatRoom(
|
|
id,
|
|
configuration.getString("password"),
|
|
Bukkit.getOfflinePlayer(
|
|
new UUID(
|
|
configuration.getLong("owner.msb"),
|
|
configuration.getLong("owner.lsb")
|
|
)
|
|
)
|
|
);
|
|
chatRoom.color = ChatColor.of(configuration.getString("color", chatRoom.color.getName()));
|
|
|
|
return chatRoom;
|
|
}
|
|
|
|
/**
|
|
* Saves a chat room to disk<br>
|
|
* A lowercase, alphanumeric id is expected
|
|
*
|
|
* @throws IOException if saving failed
|
|
*/
|
|
static void save(ChatRoom chatRoom) throws IOException {
|
|
YamlConfiguration configuration = new YamlConfiguration();
|
|
configuration.set("password", chatRoom.password);
|
|
configuration.set("color", chatRoom.color.getName());
|
|
// TODO consider just making this str to make it easier
|
|
configuration.set("owner.msb", chatRoom.owner.getUniqueId().getMostSignificantBits());
|
|
configuration.set("owner.lsb", chatRoom.owner.getUniqueId().getLeastSignificantBits());
|
|
|
|
File chatRoomFile = getFile(chatRoom.id);
|
|
configuration.save(chatRoomFile);
|
|
}
|
|
}
|